지난번 글에서 React가 뭔지에 대해서 적었으니 이번에는 React 개발환경을 어떻게 세팅하고 시작하는지에 대해 적어보려고 합니다.
create-react-app
React를 사용하려면 웹팩, 바벨 등의 설정을 해야합니다.(웹팩, 바벨이 뭔지 지금은 몰라도 됩니다.) React만 공부하기도 힘든데 웹팩, 바벨에 대해서도 공부하고 설정까지 다 직접 해야한다면 React를 시작하기도 전에 이미 지쳐버려서 공부하기를 포기해버릴지도 모릅니다. 다행히 페이스북에서 만들어준 create-react-app 을 통해 쉽게 React 프로젝트를 만들 수 있습니다.
create-react-app을 사용하는 방법은 두 가지가 있습니다.
- npm에서 create-react-app을 global로 설치하고 실행하기
- npx로 설치없이 create-react-app을 실행하기
먼저, npm으로 설치하고 실행하기입니다. 아래 명령어를 통해 create-react-app을 global로 설치합니다.
npm install -g create-react-app
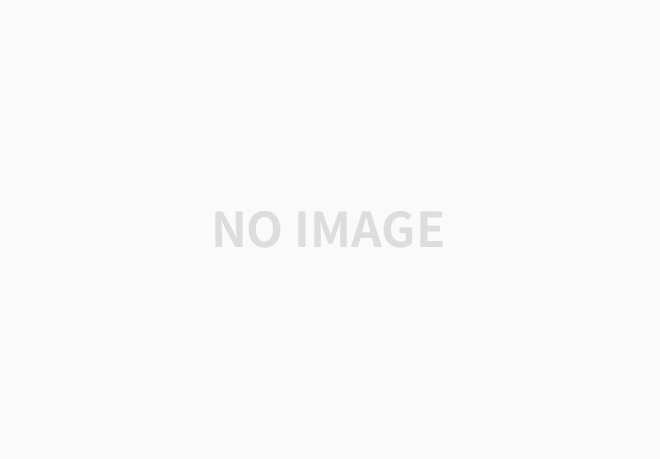
설치가 다 되었으면 React 프로젝트를 만들고 싶은 directory로 이동 후 다음 명령어로 create-react-app을 실행합니다.
create-react-app my_project
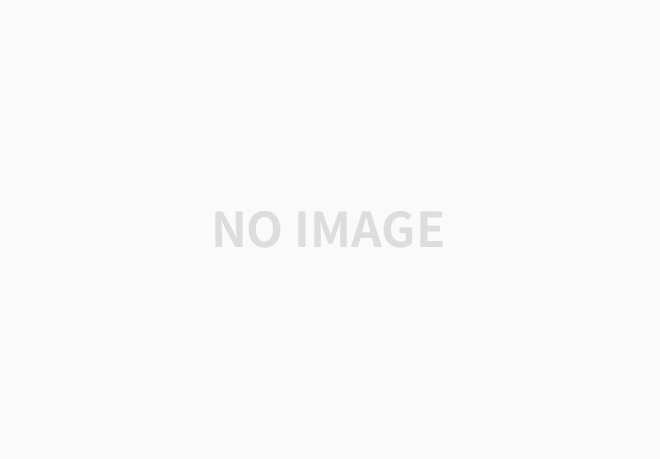
create-react-app 프로젝트명 을 입력하면 React를 사용하는데 필요한 라이브러리들과 기본 설정들이 포함된 프로젝트명 폴더가 만들어집니다.
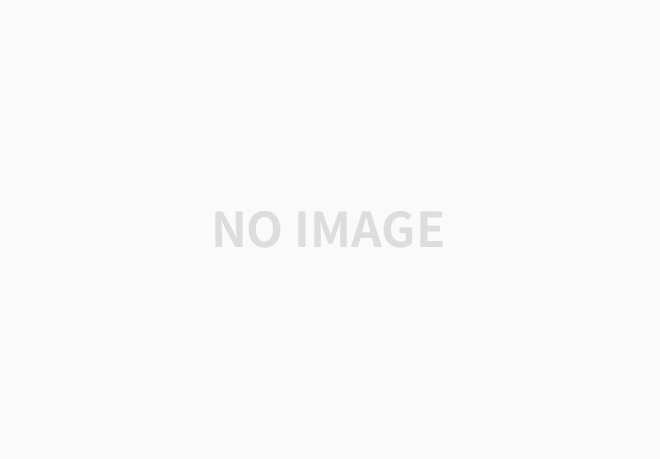
npx 명령어를 사용하면 create-react-app를 설치하지 않고 바로 실행할 수 있습니다. npx는 npm 버전이 5.2.0 이상이라면 사용가능한 명령어입니다. create-react-app이 설치되어있지 않은 상태에서 다음과 같은 명령어를 입력해도 똑같이 my_project 폴더가 만들어집니다.
npx create-react-app my_project
Inside React Project
폴더 생성이 끝났으면 내부를 살펴보겠습니다. 해당 폴더를 vscode로 열어보니 다음과 같은 파일들로 구성되어 있습니다.
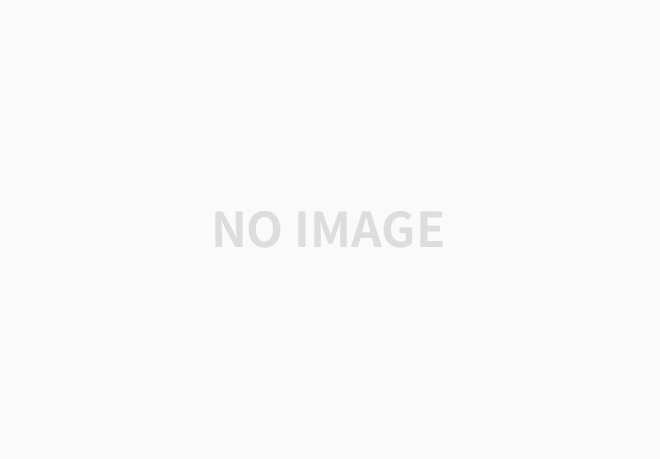
이번 글에서 눈 여겨 볼 것은 public/index.html 과 src/index.js 입니다.
먼저 index.js 입니다.
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import * as serviceWorker from './serviceWorker';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
// If you want your app to work offline and load faster, you can change
// unregister() to register() below. Note this comes with some pitfalls.
// Learn more about service workers: https://bit.ly/CRA-PWA
serviceWorker.unregister();
여기서 중요한 것은 아래의 코드입니다.
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
ReactDOM의 render 함수를 실행하는데 HTML 태그처럼 생긴 애들과 document.getElementById('root') 를 파라미터로 넘겨주고 있습니다.
- 첫 번째 파라미터는 HTML처럼 생겼지만 JSX라고 불리는 특별한 형태의 javascript 코드입니다. 무엇을 의미하는지 지금 알 필요는 없습니다.
- 두 번째 파라미터는 DOM 객체에서 가져온 id값이 root인 element입니다.
render 함수의 의미는 첫 번째 파라미터인 JSX를 두 번째 파라미터인 id가 root인 element 안에 렌더링 해주겠다는 뜻입니다.
id가 root인 element는 index.html에 들어있습니다. 아래는 index.html의 코드입니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<link rel="icon" href="%PUBLIC_URL%/favicon.ico" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<meta name="theme-color" content="#000000" />
<meta
name="description"
content="Web site created using create-react-app"
/>
<link rel="apple-touch-icon" href="%PUBLIC_URL%/logo192.png" />
<!--
manifest.json provides metadata used when your web app is installed on a
user's mobile device or desktop. See https://developers.google.com/web/fundamentals/web-app-manifest/
-->
<link rel="manifest" href="%PUBLIC_URL%/manifest.json" />
<!--
Notice the use of %PUBLIC_URL% in the tags above.
It will be replaced with the URL of the `public` folder during the build.
Only files inside the `public` folder can be referenced from the HTML.
Unlike "/favicon.ico" or "favicon.ico", "%PUBLIC_URL%/favicon.ico" will
work correctly both with client-side routing and a non-root public URL.
Learn how to configure a non-root public URL by running `npm run build`.
-->
<title>React App</title>
</head>
<body>
<noscript>You need to enable JavaScript to run this app.</noscript>
<div id="root"></div>
<!--
This HTML file is a template.
If you open it directly in the browser, you will see an empty page.
You can add webfonts, meta tags, or analytics to this file.
The build step will place the bundled scripts into the <body> tag.
To begin the development, run `npm start` or `yarn start`.
To create a production bundle, use `npm run build` or `yarn build`.
-->
</body>
</html>
아래 쪽에 보면 id가 root인 div 태그가 보입니다.
현재는 div 태그 안이 비어있지만 index.js가 실행이 되면 ReactDOM.render 함수가 실행되면서 첫 번째 파라미터인 JSX가 id가 root인 저 div 태그 안으로 들어가게 되는 것입니다.
그럼 이제 아까 만들었던 React 폴더로 이동해 React app을 실행해보자.
cd my_project
npm start
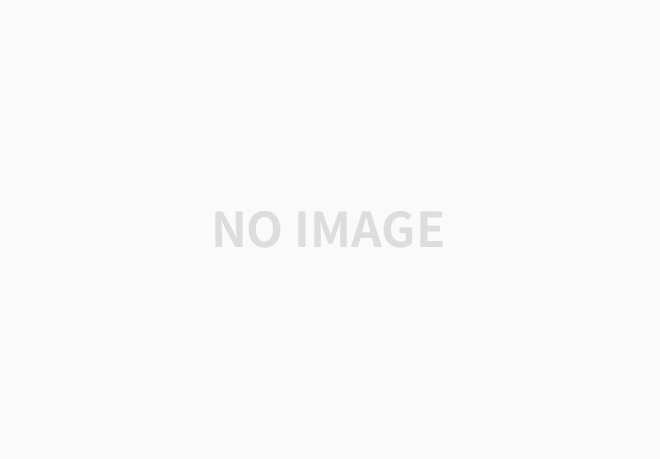
npm start 명령어가 실행 된 상태에서 http://localhost:3000 에 접속해보면 아래와 같은 화면이 뜹니다.
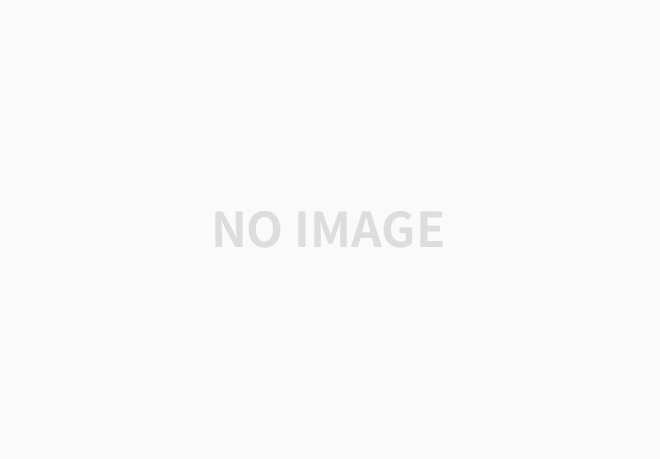
개발자 도구를 열어보면 아까 봤던 index.html 이 보이고 id가 root인 div 태그가 채워져 있는 것을 볼 수 있습니다. 아까 봤던 JSX가 HTML로 바뀌어 렌더링 된 것입니다. 다시 정리해보면
ReactDOM.render 함수는 첫 번째 파라미터를 HTML로 바꾸어 두 번째 태그 안에 넣어준다.
는 것을 알 수 있습니다.
아래와 같이 render 함수의 첫 번째 파라미터를 null로 바꾸고 저장해겠습니다. React에서는 코드 변경 사항이 생기면 자동으로 반영 되므로 새로고침을 하거나 npm start를 재실행 할 필요가 없습니다.
ReactDOM.render(
null,
document.getElementById('root')
);
그럼 예상대로 아무것도 뜨지가 않고 div 태그가 비어있게 됩니다.
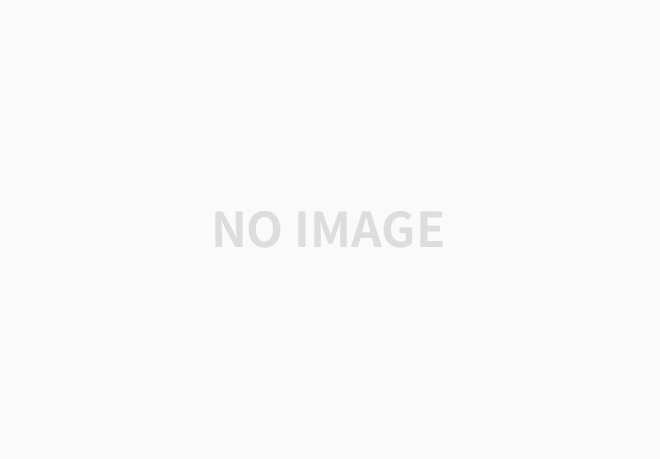
이번에는 Hello world를 띄워보겠습니다. 아래와 같이 첫 번째 파라미터에 <h1>Hello world!</h1> (완벽한 HTML이지만 이것도 사실 JSX입니다.) 를 넣고 저장해보면 Hello world가 잘 뜨는 것을 확인해볼 수 있다.
ReactDOM.render(
<h1>Hello world!</h1>,
document.getElementById('root')
);
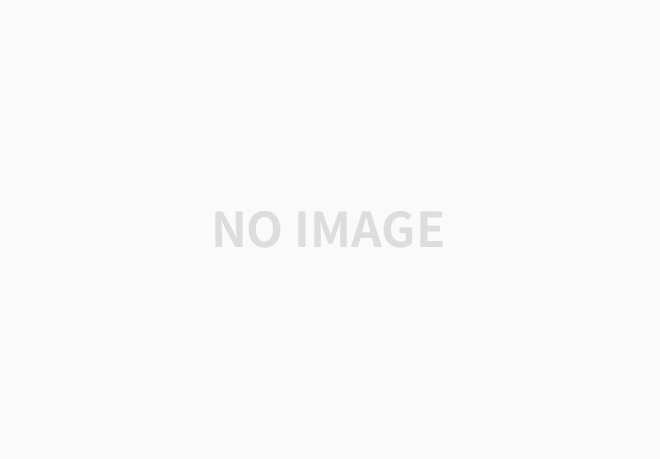
render 함수의 첫 번째 파라미터의 값을 변경해보면서 어떻게 보여지는지 확인해봤습니다.
간단히 말해 React 프로그래밍을 한다는 것은 render 함수의 첫 번째 파라미터에 들어갈 JSX를 프로그래밍 하는 것입니다.
다음 글에서는 JSX와 Virtual DOM에 대해 적어보겠습니다.
'React' 카테고리의 다른 글
VSCode에 React + ESLint + Prettier 설정하기 (0) | 2021.11.01 |
---|---|
JSX와 React Virtual DOM (0) | 2020.12.06 |
React는 무엇이고 왜 사용하는가? (0) | 2020.06.28 |